Yearly Archives: 2014
Have to catch up
Bad weekend. Due to the unexpected death of a family member i was unable to get any work done as opposed to the things i had planned. Monday was better however as i was able to both get good work done during the morning, managed to follow the lecture on the afternoon and crunch numbers during the evening. This is required as i am still not done with the first part of the assignment, although i’m getting closer by the […]
Have to catch up
Bad weekend. Due to the unexpected death of a family member i was unable to get any work done as opposed to the things i had planned. Monday was better however as i was able to both get good work done during the morning, managed to follow the lecture on the afternoon and crunch numbers during the evening. This is required as i am still not done with the first part of the assignment, although i’m getting closer by the […]
Unit Testing
So this time I’ll be blogging about what Unit testing is and how I applied it to both my projects(BST and L.List).
Generally unit testing follows your functions you’ve created and makes sure they return the correct values or just does what they are suppose to do.
The way you configure them is dependent on what your functions does.
I will state here what kind of testing my class does and how it does so.
As a whole this is my unit testing:
bool are_equal(T […]
Unit Testing
So this time I’ll be blogging about what Unit testing is and how I applied it to both my projects(BST and L.List).
Generally unit testing follows your functions you’ve created and makes sure they return the correct values or just does what they are suppose to do.
The way you configure them is dependent on what your functions does.
I will state here what kind of testing my class does and how it does so.
As a whole this is my unit testing:
bool are_equal(T […]
Unit Testing
So this time I’ll be blogging about what Unit testing is and how I applied it to both my projects(BST and L.List).
Generally unit testing follows your functions you’ve created and makes sure they return the correct values or just does what they are suppose to do.
The way you configure them is dependent on what your functions does.
I will state here what kind of testing my class does and how it does so.
As a whole this is my unit testing:
bool are_equal(T […]
Unit Testing
So this time I’ll be blogging about what Unit testing is and how I applied it to both my projects(BST and L.List).
Generally unit testing follows your functions you’ve created and makes sure they return the correct values or just does what they are suppose to do.
The way you configure them is dependent on what your functions does.
I will state here what kind of testing my class does and how it does so.
As a whole this is my unit testing:
bool are_equal(T […]
Programming – Week 3
This week I’ve learned how to create classes using header and cpp files.
This is an example of a header named Student
class Student
{
public:
Student(std::string name, int age);
int GetAge();
void SetAge(int newAge);
std::string GetName();
void SetName(std::string newName);
private:
int age;
std::string name;
};
The keyword ”public” describes what functions and variables are accesable from outside the class. All ”private” variables and functions are only accesable inside the class.
Student(std::string name, int age) is the constructor of the class. The constructor works like a […]
Programming – Week 3
This week I’ve learned how to create classes using header and cpp files.
This is an example of a header named Student
class Student
{
public:
Student(std::string name, int age);
int GetAge();
void SetAge(int newAge);
std::string GetName();
void SetName(std::string newName);
private:
int age;
std::string name;
};
The keyword ”public” describes what functions and variables are accesable from outside the class. All ”private” variables and functions are only accesable inside the class.
Student(std::string name, int age) is the constructor of the class. The constructor works like a […]
Programming – Week 3
This week I’ve learned how to create classes using header and cpp files.
This is an example of a header named Student
class Student
{
public:
Student(std::string name, int age);
int GetAge();
void SetAge(int newAge);
std::string GetName();
void SetName(std::string newName);
private:
int age;
std::string name;
};
The keyword ”public” describes what functions and variables are accesable from outside the class. All ”private” variables and functions are only accesable inside the class.
Student(std::string name, int age) is the constructor of the class. The constructor works like a […]
Programming – Week 3
This week I’ve learned how to create classes using header and cpp files.
This is an example of a header named Student
class Student
{
public:
Student(std::string name, int age);
int GetAge();
void SetAge(int newAge);
std::string GetName();
void SetName(std::string newName);
private:
int age;
std::string name;
};
The keyword ”public” describes what functions and variables are accesable from outside the class. All ”private” variables and functions are only accesable inside the class.
Student(std::string name, int age) is the constructor of the class. The constructor works like a […]
Programmering V.2
PONG!!
// main.cpp
// includes
#include
#include
#include
// Pro+: disable warning(s) that are annoying
#pragma warning(disable:4098)
// pragma directives (can also be linked through project settings)
#pragma comment(lib, ”SDL2.lib”)
#pragma comment(lib, ”SDL2main.lib”)
// structs
struct Paddle
{
float x, y;
bool input[2];
};
struct Ball
{
float x, y, xA,yA;
};
enum EGameState
{
GAME_STATE_PAUSE,
GAME_STATE_PLAY,
};
struct Game
{
SDL_Window* window;
SDL_Renderer* renderer;
int width, height;
unsigned int tick;
EGameState state;
Ball boll;
unsigned int score0, score1;
Paddle left;
Paddle right;
bool start;
};
void initialize_boll(Ball* boll, float x, float y)
{
boll->x = x;// = 502;
boll->y = y;// = 310;
boll->xA = 0.0f; //= 50;
boll->yA = 0.0f; //= -50;
}
void initialize_paddle(Paddle* paddle, float x, float y)
{
paddle->input[0] = […]
Programmering V.2
PONG!!
// main.cpp
// includes
#include
#include
#include
// Pro+: disable warning(s) that are annoying
#pragma warning(disable:4098)
// pragma directives (can also be linked through project settings)
#pragma comment(lib, ”SDL2.lib”)
#pragma comment(lib, ”SDL2main.lib”)
// structs
struct Paddle
{
float x, y;
bool input[2];
};
struct Ball
{
float x, y, xA,yA;
};
enum EGameState
{
GAME_STATE_PAUSE,
GAME_STATE_PLAY,
};
struct Game
{
SDL_Window* window;
SDL_Renderer* renderer;
int width, height;
unsigned int tick;
EGameState state;
Ball boll;
unsigned int score0, score1;
Paddle left;
Paddle right;
bool start;
};
void initialize_boll(Ball* boll, float x, float y)
{
boll->x = x;// = 502;
boll->y = y;// = 310;
boll->xA = 0.0f; //= 50;
boll->yA = 0.0f; //= -50;
}
void initialize_paddle(Paddle* paddle, float x, float y)
{
paddle->input[0] = […]
Two weeks of programming
Well, it’s been two weeks since my last post, so I’ll go through what I’ve done the past weeks.
Last week we were introduced to network programming, which I’m very interested in and have been ever since I played Diablo II with my best friend, wanting to learn how it ticked.
It isn’t my first contact with networking in practice or theory, back in high-school I went to a networking course where we learned the OSI-model among others. even if it was […]
Two weeks of programming
Well, it’s been two weeks since my last post, so I’ll go through what I’ve done the past weeks.
Last week we were introduced to network programming, which I’m very interested in and have been ever since I played Diablo II with my best friend, wanting to learn how it ticked.
It isn’t my first contact with networking in practice or theory, back in high-school I went to a networking course where we learned the OSI-model among others. even if it was […]
Two weeks of programming
Well, it’s been two weeks since my last post, so I’ll go through what I’ve done the past weeks.
Last week we were introduced to network programming, which I’m very interested in and have been ever since I played Diablo II with my best friend, wanting to learn how it ticked.
It isn’t my first contact with networking in practice or theory, back in high-school I went to a networking course where we learned the OSI-model among others. even if it was […]
Two weeks of programming
Well, it’s been two weeks since my last post, so I’ll go through what I’ve done the past weeks.
Last week we were introduced to network programming, which I’m very interested in and have been ever since I played Diablo II with my best friend, wanting to learn how it ticked.
It isn’t my first contact with networking in practice or theory, back in high-school I went to a networking course where we learned the OSI-model among others. even if it was […]
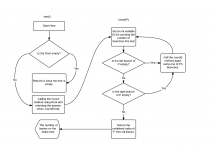
Game Programming III – Blog Post 3
Gah! I just remembered that I forgot to write this. Oh well, I’ll just get right to it, I suppose.
The main thing that was accomplished this week was the completeness of the binary search tree. While the linked list wasn’t too difficult and the logic wasn’t too strange, the binary search tree was something different. The creation of the tree was probably the easiest as it simply followed a somewhat basic logic and most of the work was to […]
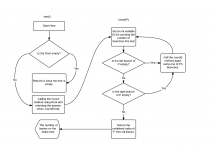
Game Programming III – Blog Post 3
Gah! I just remembered that I forgot to write this. Oh well, I’ll just get right to it, I suppose.
The main thing that was accomplished this week was the completeness of the binary search tree. While the linked list wasn’t too difficult and the logic wasn’t too strange, the binary search tree was something different. The creation of the tree was probably the easiest as it simply followed a somewhat basic logic and most of the work was to […]
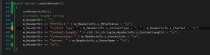
Done with the Web Server! (Week 48)
So I managed to finish my web server.
When I started coding I managed to get something up and working in a few hours and I blogged about it last week, but then I realized that there was much more to assignment 2 so I got down to business.
First of all I had to figure out how a web browser operates. Something I knew nothing about. My classmate Jonas explained how the browser sends a “GET” request (e.g. GET […]
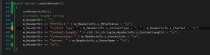
Done with the Web Server! (Week 48)
So I managed to finish my web server.
When I started coding I managed to get something up and working in a few hours and I blogged about it last week, but then I realized that there was much more to assignment 2 so I got down to business.
First of all I had to figure out how a web browser operates. Something I knew nothing about. My classmate Jonas explained how the browser sends a “GET” request (e.g. GET […]
Week 3 of Game Programming 1 – Classes
In this week of game programming, we have worked with classes. A class holds values, the amount and type of values is decided by the programmer. To learn how classes work, we had a bunch of different assignments. The assignment I chose to focus on was to make a deck of cards. I chose to focus on this because it demonstrate really well how classes work together. I made a class called Card, this class held the types Integer and String. […]
Week 3 of Game Programming 1 – Classes
In this week of game programming, we have worked with classes. A class holds values, the amount and type of values is decided by the programmer. To learn how classes work, we had a bunch of different assignments. The assignment I chose to focus on was to make a deck of cards. I chose to focus on this because it demonstrate really well how classes work together. I made a class called Card, this class held the types Integer and String. […]
Week two and three
Hello world!
The first week was easy enough, but the second week was rough, really rough. In the classes I barely understood anything the teacher went through and it took quite a while to learn and understand it. The 20 exercises that we had for the second week were difficult and took me until the middle of the third week to complete, so that put me back quite a bit. I started studying after the classes with some of the other […]
Week two and three
Hello world!
The first week was easy enough, but the second week was rough, really rough. In the classes I barely understood anything the teacher went through and it took quite a while to learn and understand it. The 20 exercises that we had for the second week were difficult and took me until the middle of the third week to complete, so that put me back quite a bit. I started studying after the classes with some of the other […]